Chapter 2: Getting started with Anaconda and Spyder
The Integrated Development Environment (IDE)
“IDE” what? Wikipedia defines it as follows:
An integrated development environment (IDE) is a software application that provides comprehensive facilities to computer programmers for software development. An IDE normally consists of at least a source code editor, build automation tools and a debugger. An IDE normally consists of at least a source code editor, build automation tools and a debugger.
en.wikipedia.org
Hence, an IDE mainly consists of a source code editor, build automation tools (e.g., integrated in a terminal/console) and a debugger.
Available are all-in-one Python-specific solutions, e.g., Spyder via Anaconda, PyCharm, Visual Studio Code and many more. You can find a broader overview here ꜛ.
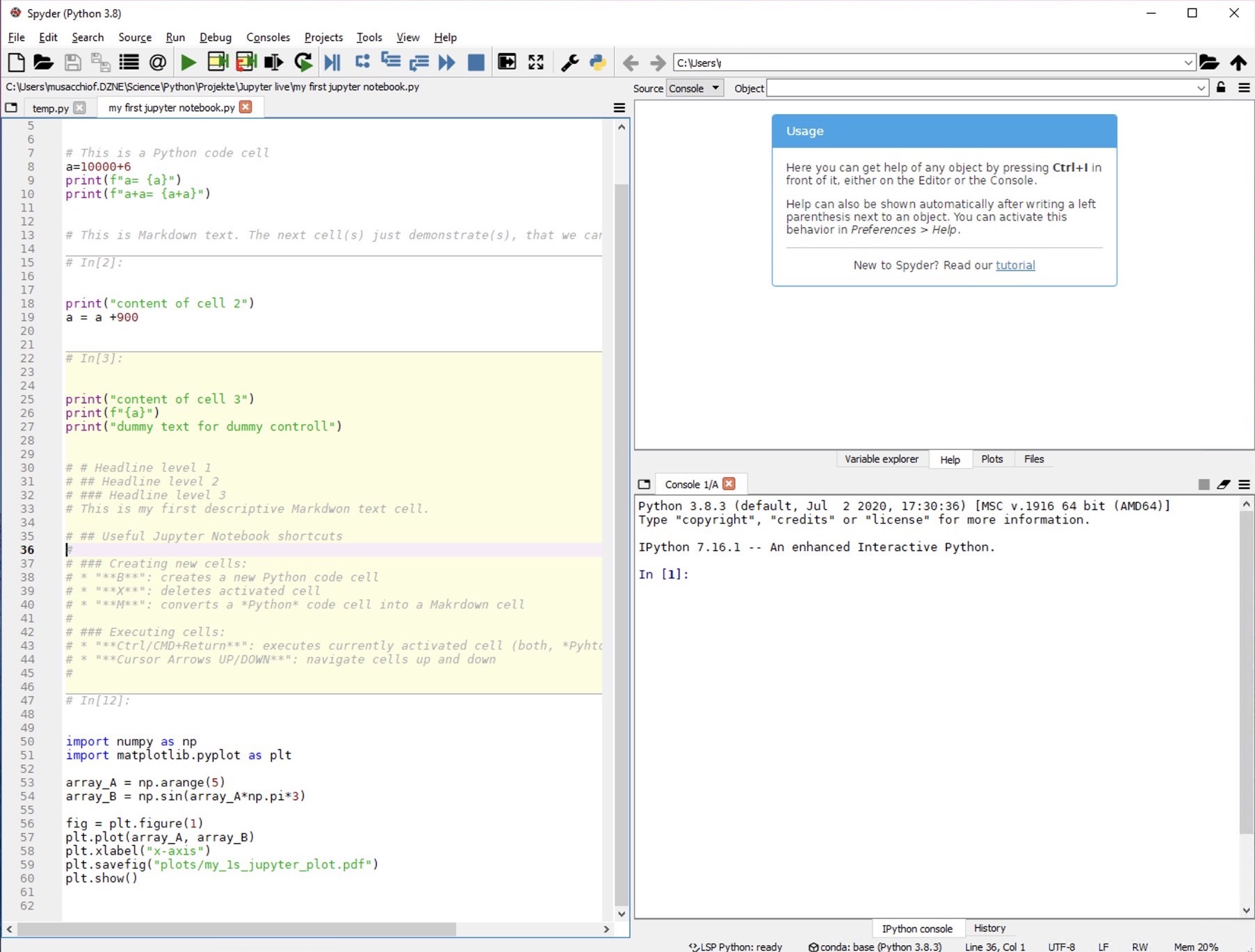
there are also online solutions like Google Colab ꜛ:
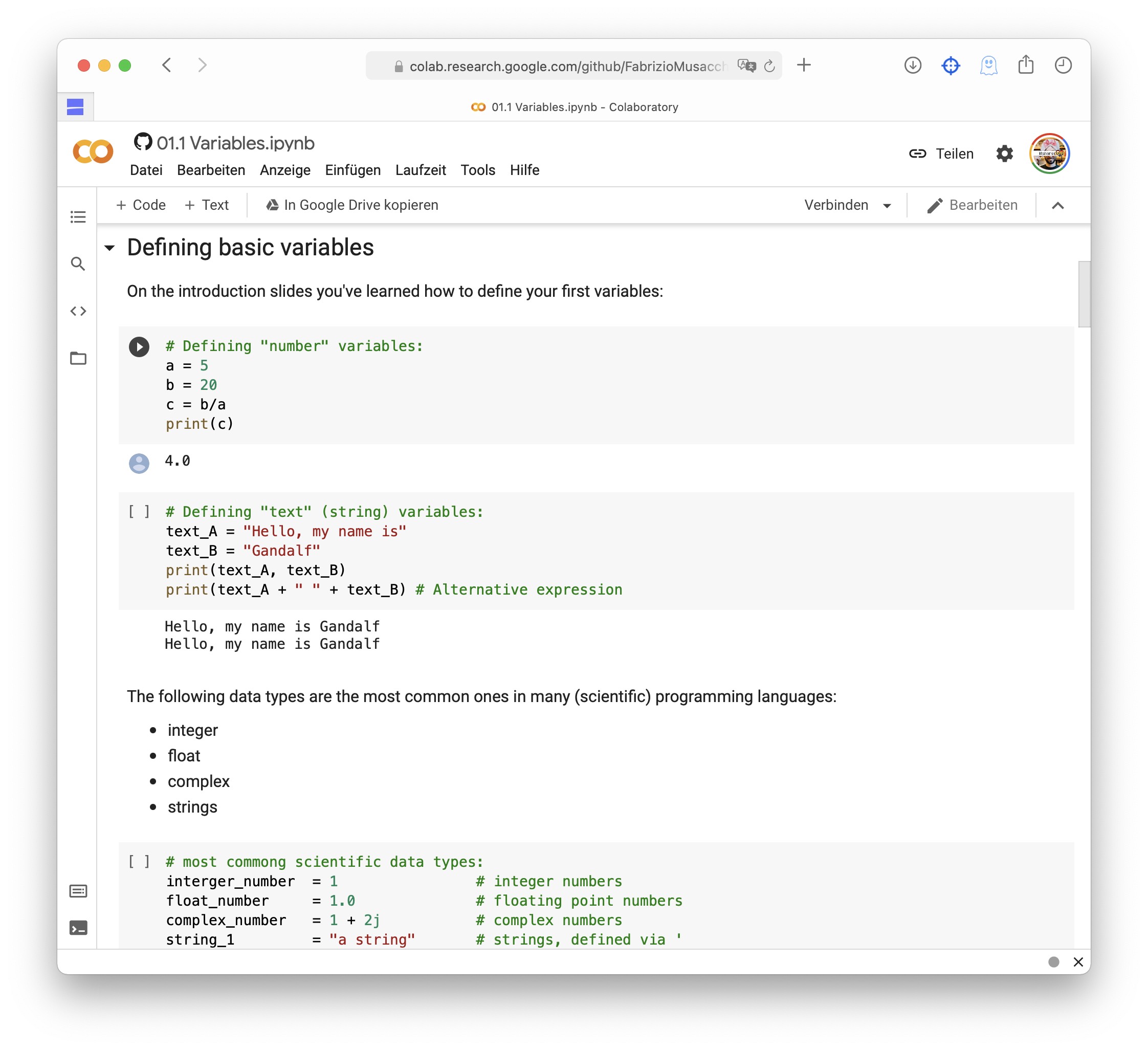
But in principle, you can use any text editor and the terminal/console of your operating system (with an installed Python version).
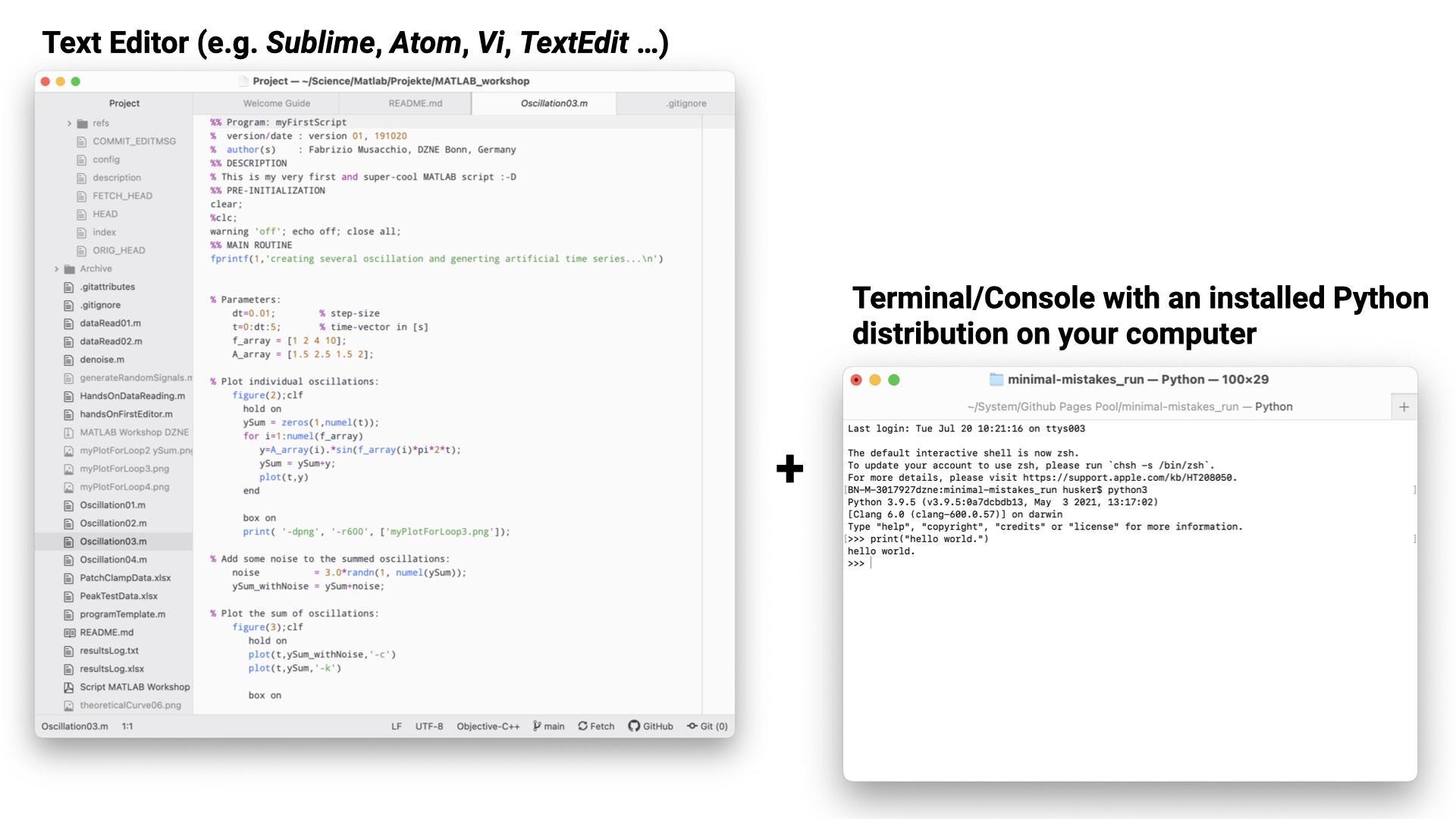
Anaconda
In this course, we will use Anaconda:
- it is free and open-source,
- comes with Python and R,
- has an easy to use editor with integrated console (Spyder),
- has a simplified package management, and
- it can be installed on MacOS, Windows and Linux.
- https://www.anaconda.com/products/individual
We will install some additional packages first, which we will need throughout our course:
Please, follow the instructions on the slides above and install the following additional packages – either during the course or in preparation of part II at home (also see voluntary homework):
- matplotlib
- numpy
- pandas
- openpyxl
- xlrd
- pingouin (requires the conda-forge channel to be added!)
Some of these packages will not be listed at first glance. If this happens, you need to add the additional channel conda-forge
. Also here, please follow the instruction on the slides
Spyder
Let’s launch Spyder:
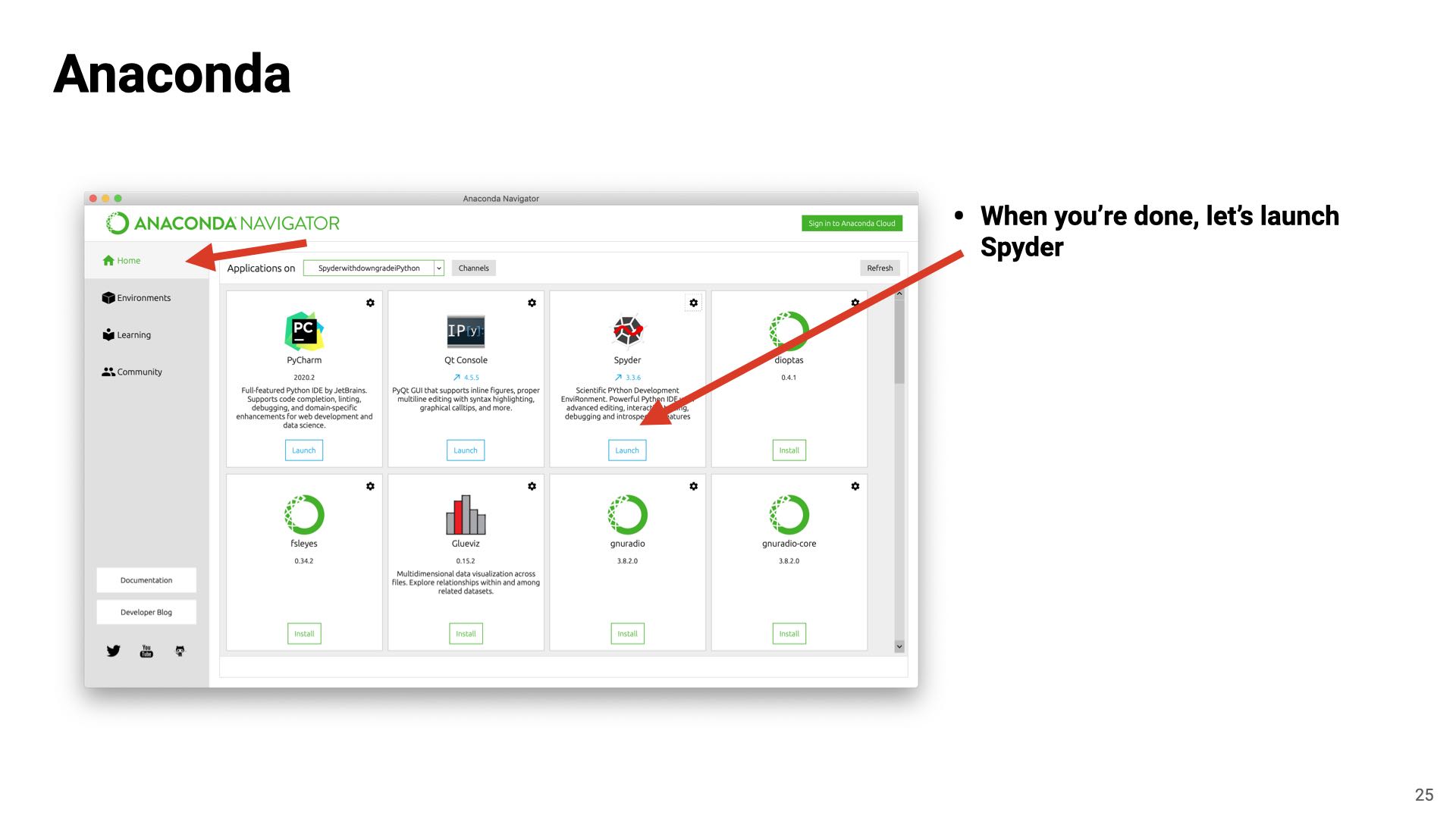
Spyder will open up and it should look like something like the screenshot:
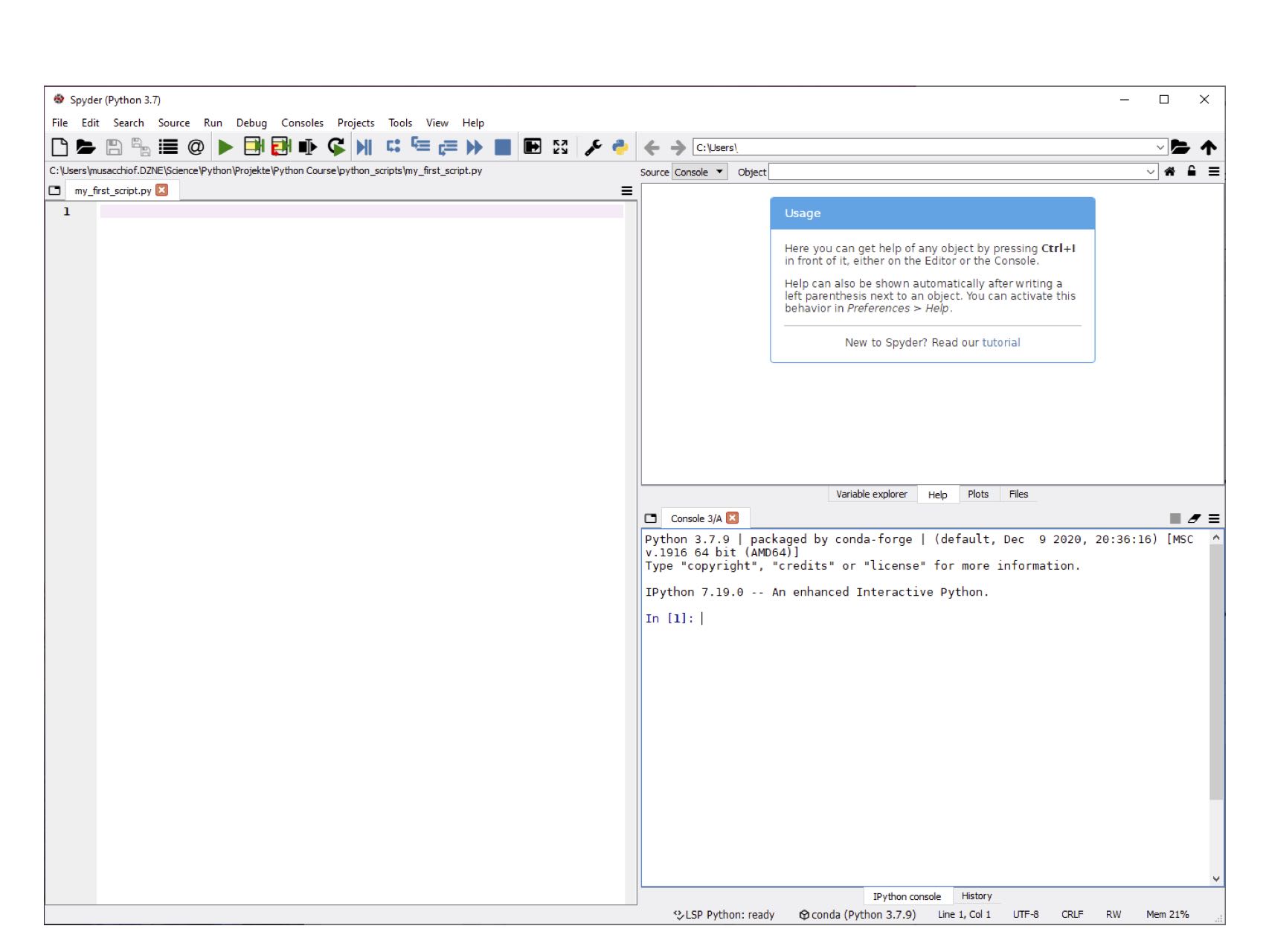
Spyder IDE explained
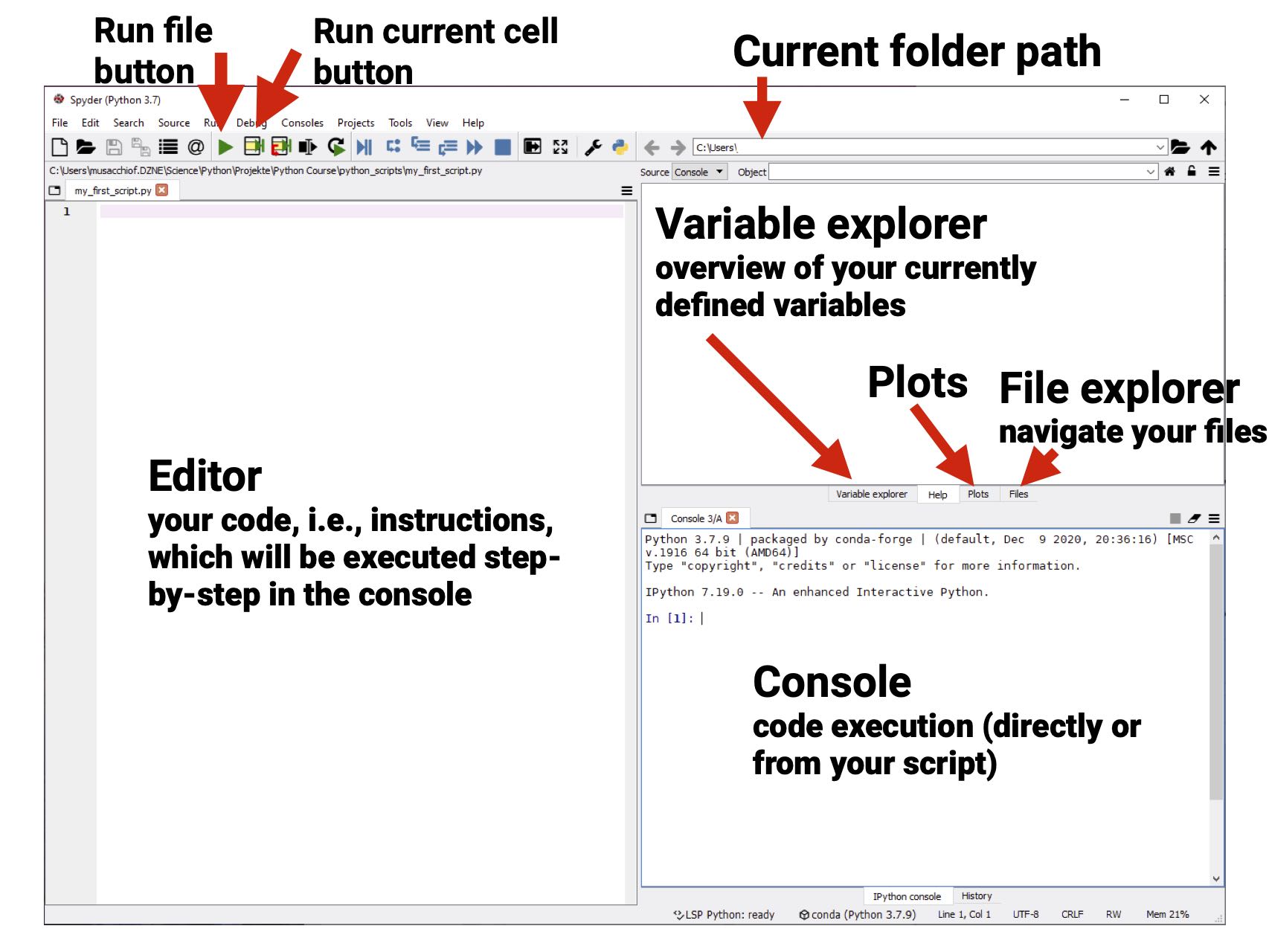
Module | Description |
---|---|
Console | code execution, either directly (by typing your commands right into this window) or from your script |
Editor | here you will write your script, in principal a text file (with the file ending “.py”), which contains line-by-line your code, that will then be executed in the console |
Variable explorer tab | an overview of all your currently defined variables |
File explorer tab | like your Windows Explorer or MacOS Finder, navigate through your files |
Plots tab | by default, all your plots are displayed in this tab |
Current folder path | view and change the current folder |
Run file button | run your script (the first execution of a new script displays a command prompt to save the script) |
Run current cell button | executes your currently selected cell (cells will be introduced later) |
Our first commands
Type your first Python commands into the console:
5+5
print("Hello world.")
Click on the Variable explorer and define your first variables in the Console:
a=5
b=10
print(a+b) # this is the default print command
print(f"a+b={a+b}") # this is a formatted print command
Type the following code into the editor and hit the Run file
button:
a=5
b=10
print(a+b)
print(b**a)
Change b
to 20
and re-run your script.
b=20
Note, that the variable b
will be updated in the Variable explorer
You can also define variables with text (“strings”):
text_A = "Hello, my name is "
text_B = "Gandalf."
print(text_A)
print(text_B)
print(text_A + text_B)
Comments
In Python, you have two options to add comments to your code:
- use
#
followed by you comment text, to add a line comment- can be placed anywhere in your script
- good for, e.g., quick annotations
- every new line needs to be initialized with a new
#
# This is a line comment placed at the line beginning:
print(f"Some text")
# Line comments can be written one after another
# and as many as you want to
print(f"Some more text") # they can be arbitrarily placed
- use
''' your block comment text '''
, to add a block comment (also called dockstring when, e.g., used within functions or classes)- good for, e.g., longer comments (it accepts line breaks within the comment block)
""" This is a block comment """
""" You can insert line breaks into block comment, which
useful e.g., for longer comments, as you
don't have to write # for each new line.
"""
General usage suggestions for comments: Where appropriate, use as many comments as possible: comment you changes and add-ons to your code, explain your code (to yourself and to colleagues and potential other users of your code), describe the units of the variables that you use, note down to-do’s and infos, if something is not working yet, etc. Your future self will thank you for that.
Code Cells
Besides comments, we can further structure our code by introducing code cell:
- use
#%%
at the beginning of a line, to introduce a new code cells - an additional
#%%
afterwards will define the code cell’s end and the beginning of a new cell - after
#%%
you can even write some text to describe the purpose of your cell - you can execute code cells cell-by-cell by hitting the
Run current cell
button (instead of always running your entire script)
#%% My first code cell
a=5
b=20
print(a+b)
print(b**a)
#%% My second code cell
text_A = "Hello, my name is "
text_B = "Gandalf."
print(text_A)
print(text_B)
print(text_A + text_B)
General suggestions for code cell: You can use code cells to separate, e.g., the variable definition part, your main algorithm and a potential plot command section from each other.
Summary
After all these first commands, your Spyder window might look similar to the following screenshot:
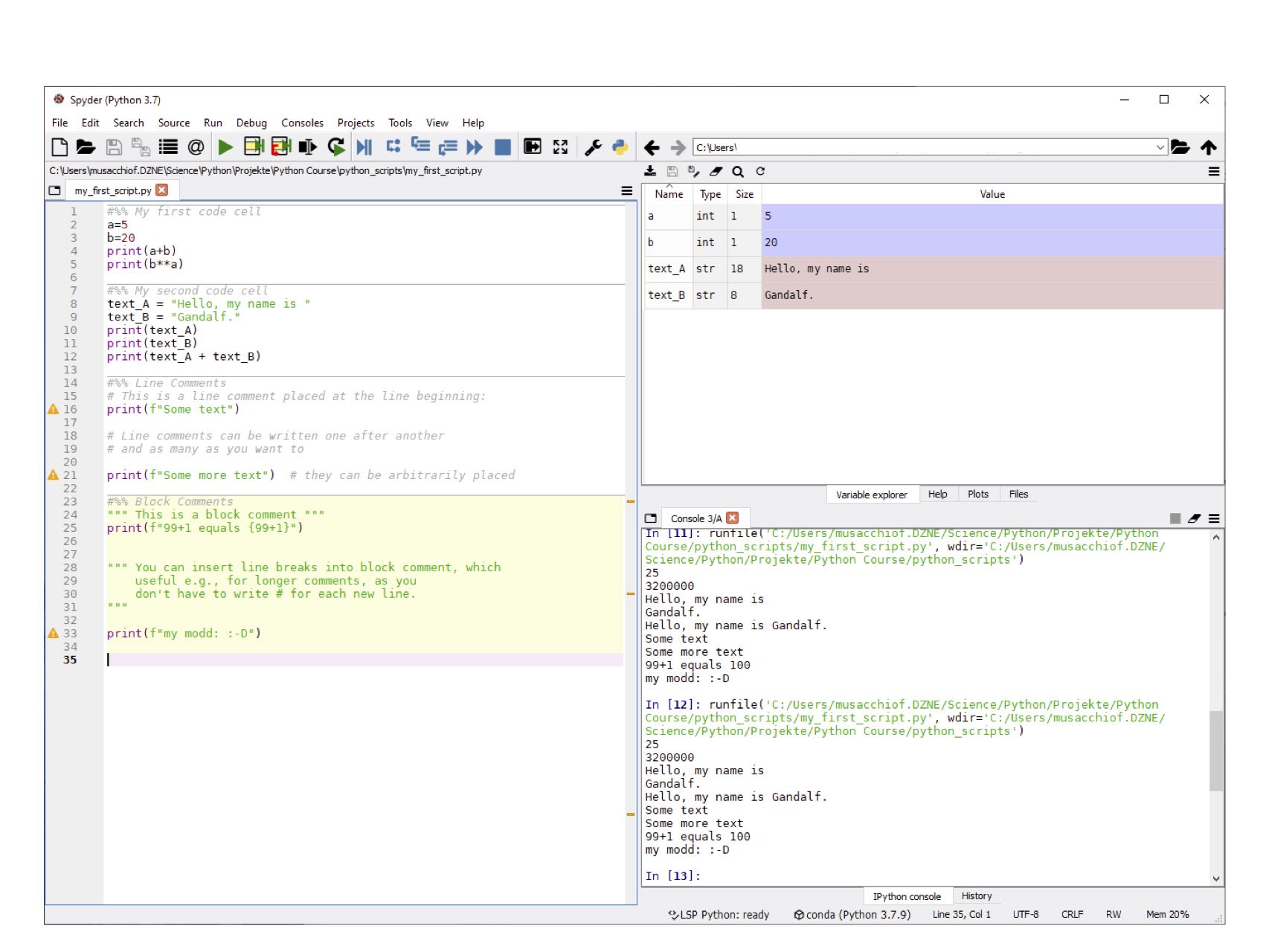